 | 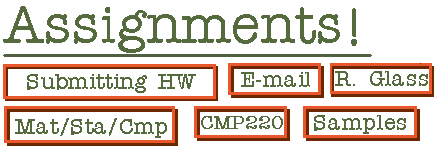 |
|
|
Assignments Revised: |
| Number | Assignment | Due Date |
|
|
| 1 |
Read
The Cuckoos' Egg by Clifford Stoll
|
3/1 |
|
|
| 2 |
Research internal floating point format and algorithims to convert to and from binary. Use it to show the binary representation of either 3.14 or 2.71
|
2/18 |
|
|
| 3 |
Write a Java or C++ program that will byte swap 16 bites from a data file. Using notepad, create a file containing AB. The program must read this file
data into an integer. From the value of the integer (HINT: 16706 or 16961) and write out the integer data in another file. Using notepad, verify that
the file contains BA.
|
3/4 |
|
|
| 4 |
Write two programs. One called encode and the other called decode that will write and read respectively a file of (16 bit) integers (binary) that are codewords as defined for the Hamming code in the textbook. All operations are to be done with bitwise operators.
Encode | Decode |
- Reads a character until until Newline is ecountered.
- Creates Hamming codeword with even parity.
- Write the codeword integers to a data file of integers.
|
- While not eof on the file of codewords.
- Read a codeword.
- If error then correct.
- Recover character.
- Print
This program must also read and decode the data file created with the encode but also the data file containing your codeword message. (Click here to get your Data file containing the SECRET MESSAGE). The file unknown.exe is a self extracting DOS executable archive file. Download this archive file to a directory (folder) on your machine and execute by either a double-click or choosing START-RUN option. At this point, you should find several files including a readme.txt.
|
The low order bit is the position 1 of the code word. Bits 13 - 16 are unused.
Programming considerations
- Programs MUST perform all operations on the integer codeword. The integer cannot be unpacked into an array.
Bitwise Operators | C |
And | & |
Or | | |
Exclusive Or | ^ |
Left Shift | << |
Right Shift | >> |
Ones' Complement | ~ |
I/O | C |
|
See cinfo.htm
|
- Grading will also be based on coding style. Use of functions and procedures that perform simple tasks (such as build a codeword given a character) is required.
Available now are very simple programs, in C and Pascal that will do nothing more than read and write a file of integers. , See how a file written by one language CAN ACTUALLY READ a file created by another language (if you know the format). ACT NOW!!! (download here) Be the very first in your class to own these very simple programs. Come visit:
http://www.matcmp.sunynassau.edu/~glassr/assign/cmp220
Watch this page for further updates.
|
3/18 |
|
|
| Final |
- Write the following program in the asdsmebly language defined in the handout:
SUM = 0
FOR I = 0, I < 10, I = I + 1
SUM = SUM + I
- Assemble the program you wrote in the previous part. Assume that variable adddress begin at address 1000 and they are 12 bit addressable.
- For each unique statement of the program
in the previous parts, trace the statements of the fetch / decode and execute cycle
defined in the handout. Be sure to state what regisiters are changed and what their new vaules are. Unique means that only trace
one occurance of a particular statement. For example, if your program contains two ADDD instructions, you only need trace one. If an ADDD
is executed several time, you only need trace one.
- Write the binary versions of the microcode instructions you used in the previous part. For example, you need to write the binary
(only once) for the statement PC + PC + 1; rd
|
5/20 |
|
|